C Program To Implement Double Ended Queue Using Linked List
Posted By admin On 06.02.20- C Program To Implement Double Ended Queue Using Linked Listings
- Double Ended Queue In C
- C Program To Implement Double Ended Queue Using Linked Listing
- Double Ended Queue Using Array In C++
- C Program To Implement Double Ended Queue Using Linked List
- Stack, Bracket checker program, Polish Notations infix to postfix conversion, FIFO Queue, Circular Queue, Double Ended Queue, Linked List - Linear, double and Circular - all operations, Stack and Queue using Linked List; What is stack, algorithms for Push and Pop operation. Implementation of Stack data structure using C.
- Linked list is a data structure consisting of a group of nodes which together represent a sequence. Here we need to apply the application of linkedlist to perform basic operations of queue. Here is source code of the C Program to implement queue using linked list. The C program is successfully compiled and run on a.
C Program To Implement Double Ended Queue Using Linked Listings
It's an exercise from CLRS 3rd:
Implementation of Deque using doubly linked list Deque or Double Ended Queue is a generalized version of Queue data structure that allows insert and delete at both ends. In previous post Implementation of Deque using circular array has been discussed. /* c++ program to implement double ended queue using doubly linked list with.
10.2-3 Implement a queue by a singly linked list L. The operations ENQUEUE and DEQUEUE should still take O(1) time.
It's not hard to implement a queue using a singly linked list. My problem is about the time complexity. How to implement ENQUEUE and DEQUEQUE that take O(1)?
Dequeue using linked list in C. Get all solutions of any problem related to Programming in C,JAVA language.
What I found on google is something like using pointers to track both head and tail. Now the problem becomes how to track head and tail on a singly linked list using O(1) time? IMHO it takes O(n) to track the tail. Am I right?
3 Answers
It will take O(1) time to manage the head and tail pointers.
Enqueue:
Yaminabe Aries - Tail Tale 'It. It would be an honor to be killed by your cooking, Mistress Rumi!' For quite some time now, the wonderful folks over at wildcritters.us have been working on translating Yaminabe Aries Tail Tale. For those who don't know, it's a Japanese visual novel released in 2000. It was released in limited numbers, and has. Yaminabe aries tail tale english downloading. Visual Novels 24067 Releases 58764 Producers 8223 Characters 77934 Staff 17125 VN Tags 2382 Character Traits 2472 Users 140679 Threads 11094 Posts 120598.
Dequeue:
Note: You will also have to perform bounds checking and memory allocation / de-allocation before carrying out pointer assignments
Abhishek BansalAbhishek Bansalit is easy, simply enque at the end and deque at the front, and setup 2 pointer(or unique_ptrs) pointing to end and front, that will do. like this:
Above is only a demonstration code, yet you can see that you don't need to iterate through the entire list to enque or deque.
std::list
is what you're looking for if you're allowed to use std
containers.
If not (I assume that's the case), try answering the question: why do you need to perform n operations? Can you just store the pointer to the end?
Smash bros reddit. Say, you have a signly linked list and a pointers head
and tail
A list item has next
pointer.
- If you enqueue a new item, you just add a new item, amend the former 'first' item's
next
pointer and repoint thehead
pointer to the new item. That's 3 operations = O(1) - If you dequeue an item, you move the
last
pointer to the one pointed on by the last item'snext
pointer` and delete the item - 2 operations = O(1)
Double Ended Queue In C
DarkWandererNot the answer you're looking for? Browse other questions tagged c++calgorithmdata-structures or ask your own question.
In this tutorial you will learn about double ended queue (dequeue) in C.
What is Dequeue?
Also Read: Circular Queue in C
C Program To Implement Double Ended Queue Using Linked Listing
Operations on a Dequeue
Double Ended Queue (Dequeue) in C
2 4 6 8 10 12 14 16 18 20 22 24 26 28 30 32 34 36 38 40 42 44 46 48 50 52 54 56 58 60 62 64 66 68 70 72 74 76 78 80 82 84 86 88 90 92 94 96 98 100 102 104 106 108 110 112 114 116 118 120 122 124 126 128 130 132 134 136 138 140 142 144 146 148 150 152 154 156 158 160 162 164 166 168 170 172 174 176 178 180 182 184 186 188 190 192 194 196 198 200 202 | #include<process.h> { intrear,front; intempty(dequeue*p); voidenqueueR(dequeue*p,intx); intdequeueF(dequeue*p); voidprint(dequeue*p); voidmain() inti,x,op,n; { printf('n1.Createn2.Insert(rear)n3.Insert(front)n4.Delete(rear)n5.Delete(front)'); printf('n6.Printn7.ExitnnEnter your choice:'); { scanf('%d',&n); printf('nEnter the data:'); for(i=0;i<n;i++) scanf('%d',&x); { exit(0); enqueueR(&q,x); break; case2:printf('nEnter element to be inserted:'); { exit(0); break; case3:printf('nEnter the element to be inserted:'); { exit(0); break; case4:if(empty(&q)) printf('nQueue is empty!!'); } x=dequeueR(&q); break; case5:if(empty(&q)) printf('nQueue is empty!!'); } x=dequeueF(&q); break; case6:print(&q); } } voidinitialize(dequeue*P) P->rear=-1; } intempty(dequeue*P) if(P->rear-1) } intfull(dequeue*P) if((P->rear+1)%MAXP->front) } voidenqueueR(dequeue*P,intx) if(empty(P)) P->rear=0; P->data[0]=x; else P->rear=(P->rear+1)%MAX; } { { P->front=0; } { P->data[P->front]=x; } intdequeueF(dequeue*P) intx; x=P->data[P->front]; if(P->rearP->front)//delete the last element else } intdequeueR(dequeue*P) intx; x=P->data[P->rear]; if(P->rearP->front) else } voidprint(dequeue*P) if(empty(P)) printf('nQueue is empty!!'); } inti; { i=(i+1)%MAX; } |
2.Insert(rear)
3.Insert(front)
4.Delete(rear)
Double Ended Queue Using Array In C++

6.Print
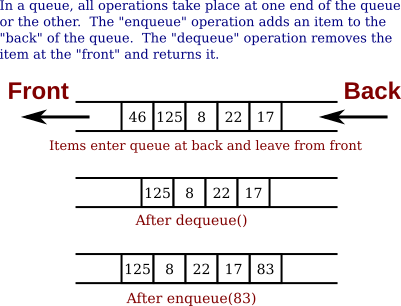
7.Exit
Enter your choice:1
Enter number of elements:3
Enter the data:4 6 7
1.Create
2.Insert(rear)
3.Insert(front)
4.Delete(rear)
5.Delete(front)
6.Print
7.Exit
Enter your choice:6
4
6
7
1.Create
2.Insert(rear)
3.Insert(front)
4.Delete(rear)
5.Delete(front)
6.Print
7.Exit
Enter your choice:4
Element deleted is 7
1.Create
2.Insert(rear)
3.Insert(front)
4.Delete(rear)
5.Delete(front)
6.Print
7.Exit
Enter your choice:7