Java Serial Port Read Write Example
Posted By admin On 22.12.19I have modified the example shown on https://code.google.com/p/java-simple-serial-connector/wiki/jSSC_examples to show read/write from java program. I can run the program, however the data I send using serialPort.writeString('HelloWorld'); does not seem to be read in the SerialPortReader event class. Could any one please point what the issue is ?
An RS-232 serial communications port. SerialPort describes the low. Class java.lang.Object. Arrive at the serial port. Even if the user doesn't read the data. Read from a Serial port, notifying when data arrives: COM Port « Development Class « Java. * to hold a conversation. */ protected void converse() throws IOException { System.out.println('Ready to read and write port.' Open a serial port using Java Communications: 5.
}
AyubAyub3 Answers
You can't read data from the same port where you write(COM1 here). I have followed the below steps for reading and writing using JSSC.
Fake your serial port with SerialPortMonitor.
Send data from COM2 from the SerialPortMonitor device installed.
Mode->Spy would show your written string 'HelloWorld' and received String 'OK'
Make the below modifications and check your code:
The port reader class: (You were missing the Override annotation and passing in the serial port)
The command
sends the string
HelloWorld
from COM1. The SerialPortReader class that you are implementing causes COM1 to listen for the event type isRXCHAR (AKA when COM1 receives a char).
Do you have a serial cable connected to the serial port?
Unless you cross the RX and TX pins of COM1 or have a separate COM port (whose TX and RX is connected to COM1's RX and TX pins respectively), the SerialPortReader will never be activated.
if your device doesn't require RTS/CTS flow controlor you dont have a fully connected serial cable
( only RX, TX, GND)
you should switch off the data terminal ready (dtr)signal for the serial communication
add this
after
Not the answer you're looking for? Browse other questions tagged javaserial-port or ask your own question.
If you would like to use the jSerialComm library in a completely asynchronous fashion, you can enable event-based callbacks via the addDataListener() method. This method takes your own custom callback object as a parameter which must implement the SerialPortDataListener interface. In any of the event-driven callback modes, you can register your own custom callback to be triggered upon certain serial I/O events.
The events you can listen for are:
- Data Available for Reading (LISTENING_EVENT_DATA_AVAILABLE)
- Data Writing Successful (LISTENING_EVENT_DATA_WRITTEN)
- Data Bytes Received (LISTENING_EVENT_DATA_RECEIVED)
- Fixed-Length Data Packet Received
- Byte- or Multibyte-Delimited Message Received
Data Available for Reading Example
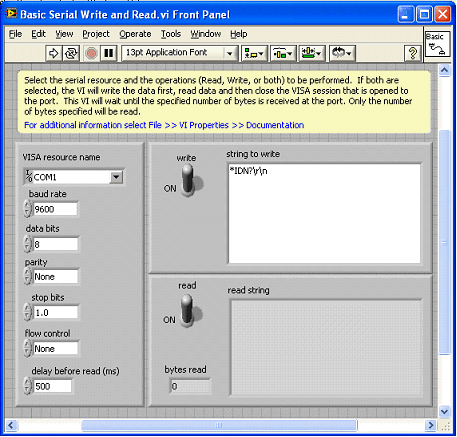
In this case, your callback will be triggered whenever there is any data available to be read over the serial port. Once your callback is triggered, you can optionally call bytesAvailable() to determine how much data is available to read, and you must actually read the data using any of the read()
or readBytes()
methods.
The following example shows one way to use this event trigger:
Data Writing Successful Example
In this case, your callback will be triggered whenever all data you have written using any of the write()
or writeBytes()
methods has actually been transmitted.
Note that this mode of operation is only functional on Windows operating systems due to limitations in Linux's handling of serial writes.
Read Right
The following example shows one way to use this event trigger:
Data Bytes Received Example
In this case, your callback will be triggered whenever some amount of data has actually been read from the serial port. This raw data will be returned to you within your own callback, so there is no further need to read directly from the serial port.
The following example shows one way to use this event trigger:
However, here is a simpler implementation for Excel: =FORECAST( NewX,OFFSET( KnownY,MATCH( NewX, KnownX,1)-1,0,2), OFFSET( KnownX,MATCH( NewX, KnownX,1)-1,0,2)) To use it either: • Copy the formula above into Excel and replace KnownX and KnownY with the cell reference for the tabulated x and y values and NewX with the x-value to interpolate, OR • Define names for the KnownX and KnownY ranges ( Insert→Name→Define in Excel 2003) and replace NewX with the x-value to interpolate. Implementing Interpolation with Microsoft Excel The linear interpolation equation above can be implemented directly in Microsoft Excel provided the tabulated values are monotonic in x, that is the x-values are sorted and no two are equal. You can download the Excel linear interpolation example. The online has the full 6 line implementation along with a good explanation of how it works.
Fixed-Length Data Packet Received
In this case, your callback will be triggered whenever a set fixed amount of data has been read from the serial port. This raw data will be returned to you within your own callback, so there is no further need to read directly from the serial port.
Note that in this case, you must implement the SerialPortPacketListener which is itself a sub-class of the SerialPortDataListener.
C# Serial Port Write Bytes
The following example shows one way to use this event trigger. Notice that in this example, we actually define a qualified listener class instead of passing in an anonymous one as in the previous examples. Either method is fine, and both are shown simply for illustrative purposes:
Byte- or Multibyte-Delimited Message Received
In this case, your callback will be triggered whenever a message has been received based on a custom delimiter that you specify. This delimiter can contain one or more consecutive bytes and can indicate either the beginning or the end of a data packet. The raw data will be returned to you within your own callback, so there is no further need to read directly from the serial port.
Note that in this case, you must implement the SerialPortMessageListener which is itself a sub-class of the SerialPortDataListener.
The following example shows one way to use this event trigger. Notice that in this example, we actually define a qualified listener class instead of passing in an anonymous one as in the previous examples. Either method is fine, and both are shown simply for illustrative purposes: